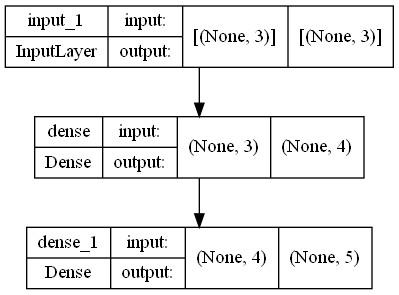
Hello everyone
Recently thought of
How to display the deep learning model in python's keras?
How about making this model structure more intuitive and understandable?
Just use graphviz
After installing graphviz
According to the following program example, the keras model architecture diagram can be generated
# Tensorflow needs to be installed import os import tensorflow as tf from tensorflow.keras.utils import plot_model # Add an input layer inputs = tf.keras.Input(shape=(3,)) # Add a new dense layer and put the dense layer behind the input layer x = tf.keras.layers.Dense(4, activation=tf.nn.relu)(inputs) # Add another dense layer and put the dense layer behind the previous dense layer # The final output is the outputs data outputs = tf.keras.layers.Dense(5, activation=tf.nn.softmax)(x) # Put the head and tail layers of the model into the Model parameter and package them into a complete model model = tf.keras.Model(inputs=inputs, outputs=outputs) os.environ["PATH"] += os.pathsep + 'C:\graphviz-2.44.1-win32/Graphviz/bin/' # path to install graphviz # generate model image plot_model( model, # model object to_file='model_.png', # The picture to be stored show_shapes=True, # show input and output dimensions show_layer_names=True # show layer names )
The picture is similar to this
Dimensions from input layer to output layer
Everything is very clear
This is also a very important parameter of the model in deep learning
Unfortunately, this method cannot be used in pytorch
For your reference
Message Board
Feel free to leave suggestions and share! Thanksgiving!